Keycloak is a powerful open-source identity and access management solution that supports single sign-on (SSO), user federation, and social login integration. NestJS, on the other hand, is a progressive Node.js framework designed to build scalable and maintainable server-side applications.
Integrating Keycloak with NestJS can help you secure your application effectively while leveraging modern development practices. This article will walk you through implementing Keycloak in a NestJS application step by step.
Why Use Keycloak with NestJS?
Keycloak provides robust features for user authentication and authorization, which can be seamlessly integrated into a NestJS application. Here’s why you should consider it:
- Comprehensive Authentication: Handles SSO, social login, and more.
- Centralized User Management: Manage users and roles from a unified dashboard.
- Security: Implements OAuth2 and OpenID Connect for secure communication.
- Ease of Integration: Keycloak adapters simplify adding authentication to your backend.
Getting Started with Keycloak and NestJS
To integrate Keycloak with NestJS, you need the following prerequisites:
- Node.js and npm: Ensure you have Node.js (v12 or higher) installed.
- NestJS Application: A functional NestJS application.
- Keycloak Server: A running instance of Keycloak.
Step 1: Setting Up Keycloak
- Download and Install Keycloak:
- Visit the Keycloak website and download the latest version.
- Extract and start the Keycloak server using:
bash
CopyEdit
./bin/standalone.sh
- Create a Realm:
Realms are Keycloak’s organizational units. Create a new realm for your application in the admin console. - Add a Client:
- Navigate to the “Clients” section in your realm.
- Click on “Create” and configure your client. Set Access Type to confidential for server-side applications.
- Create Roles and Users:
- Add roles that your application will use.
- Create users and assign them roles.
Step 2: Install Required Dependencies
In your NestJS application, install the required dependencies to integrate Keycloak:
bash
CopyEdit
npm install @nestjs/passport passport-keycloak-oauth2 passport
npm install –save-dev @types/passport-keycloak-oauth2
These packages will help you implement Keycloak’s OAuth2 strategy in your application.
Step 3: Configure the Keycloak Module
Create a keycloak.module.ts file to encapsulate Keycloak configuration in your application.
typescript
CopyEdit
import { Module } from ‘@nestjs/common’;
import { PassportModule } from ‘@nestjs/passport’;
import { KeycloakStrategy } from ‘./keycloak.strategy’;
@Module({
imports: [PassportModule],
providers: [KeycloakStrategy],
})
export class KeycloakModule {}
Step 4: Implement Keycloak Strategy
The Keycloak strategy defines how your application handles authentication.
typescript
CopyEdit
import { PassportStrategy } from ‘@nestjs/passport’;
import { Strategy } from ‘passport-keycloak-oauth2’;
import { Injectable } from ‘@nestjs/common’;
@Injectable()
export class KeycloakStrategy extends PassportStrategy(Strategy) {
constructor() {
super({
clientID: ‘your-client-id’,
clientSecret: ‘your-client-secret’,
realm: ‘your-realm’,
authServerURL: ‘http://localhost:8080/auth’,
callbackURL: ‘http://localhost:3000/auth/callback’,
});
}
async validate(accessToken: string): Promise<any> {
// Validate and return user information
return { accessToken };
}
}
Replace your-client-id, your-client-secret, and other placeholders with values from your Keycloak setup.
Step 5: Protect Endpoints Using Guards
NestJS guards are used to secure application routes. Create a custom guard for Keycloak.
typescript
CopyEdit
import { CanActivate, ExecutionContext, Injectable } from ‘@nestjs/common’;
import { AuthGuard } from ‘@nestjs/passport’;
@Injectable()
export class KeycloakAuthGuard extends AuthGuard(‘keycloak-oauth2’) implements CanActivate {
canActivate(context: ExecutionContext): boolean {
return super.canActivate(context) as boolean;
}
}
Use this guard in your controllers to protect specific endpoints.
Step 6: Integrate Keycloak in a Controller
Here’s an example of a protected route:
typescript
CopyEdit
import { Controller, Get, UseGuards } from ‘@nestjs/common’;
import { KeycloakAuthGuard } from ‘./keycloak-auth.guard’;
@Controller(‘protected’)
export class ProtectedController {
@Get()
@UseGuards(KeycloakAuthGuard)
getProtectedData() {
return { message: ‘This route is protected by Keycloak!’ };
}
}
Any attempt to access this route without proper authentication will be denied.
Step 7: Testing Your Integration
- Start the Keycloak server.
- Run your NestJS application.
- Access the protected route via a browser or API client. You should be redirected to Keycloak for authentication.
Best Practices for Keycloak Integration
- Environment Variables: Store sensitive data like client secrets in environment files.
- Role-Based Access Control (RBAC): Leverage roles to define fine-grained permissions.
- Secure Callback URLs: Ensure the callback URL is protected against unauthorized access.
- Token Validation: Validate JWT tokens to enhance security.
Advantages of Using Keycloak
- Scalability: Handles large user bases with ease.
- Flexibility: Supports a wide range of authentication protocols.
- Ease of Use: Comprehensive admin console for managing users and roles.
Challenges and Solutions
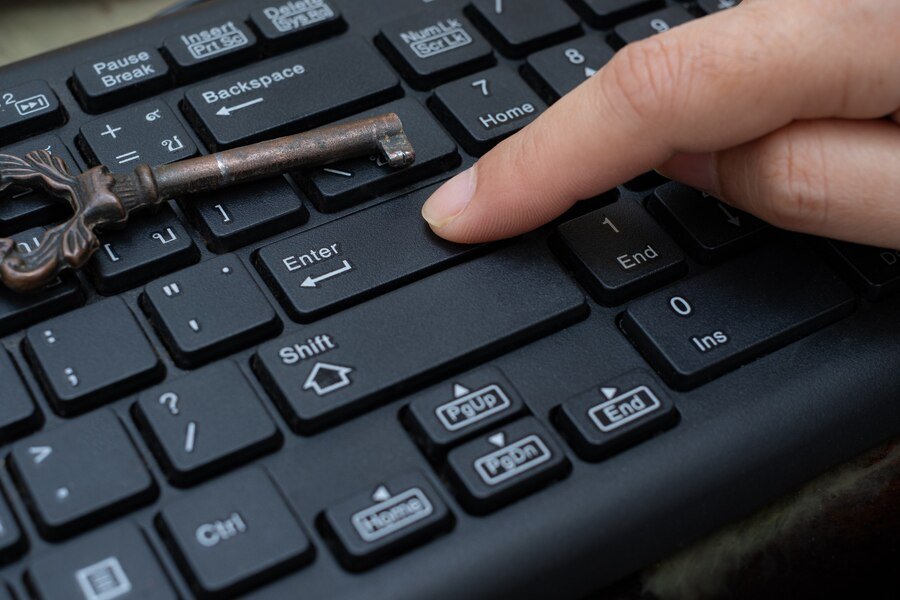
- Configuration Complexity:
Use clear documentation and step-by-step guides like this one to simplify setup. - Debugging Issues:
Enable detailed logging in both Keycloak and your application to troubleshoot effectively. - Performance Concerns:
Optimize Keycloak configurations and scale your deployment as needed.
Conclusion
Integrating Keycloak with NestJS is a powerful way to secure your application with modern authentication protocols. By following the steps outlined in this guide, you can create a robust, secure, and scalable application.
FAQs
Can I use Keycloak with other frameworks?
Yes, Keycloak supports various frameworks, including Spring Boot, Express, and more.
Is Keycloak free to use?
Yes, Keycloak is open-source and free to use under the Apache 2.0 license.
How do I integrate social logins with Keycloak?
Keycloak allows you to configure social logins through its admin console under “Identity Providers.”
Can I deploy Keycloak on the cloud?
Yes, Keycloak can be deployed on platforms like AWS, Azure, and Google Cloud.
Does Keycloak support multi-factor authentication (MFA)?
Yes, Keycloak has built-in support for MFA to enhance security.