WebSockets have revolutionized real-time communication on the web by enabling bidirectional, full-duplex connections between clients and servers. Among the many WebSocket libraries available, Gorilla WebSocket stands out as one of the most popular and reliable solutions for the Go (Golang) programming language. This article explores what Gorilla WebSocket is, its key features, how it works, and how to implement it in a Go application.
What is Gorilla WebSocket?
Gorilla WebSocket is an open-source WebSocket library for Go that provides an efficient and easy-to-use API for managing WebSocket connections. It is designed for building real-time applications, such as chat systems, stock market dashboards, online gaming platforms, and IoT (Internet of Things) applications.
Why Use Gorilla WebSocket?
Gorilla WebSocket is widely used because of its:
- Performance: It efficiently handles thousands of concurrent WebSocket connections.
- Reliability: It follows the WebSocket standard (RFC 6455) and provides a stable, production-ready solution.
- Ease of Use: It simplifies WebSocket implementation in Go applications.
- Flexibility: It allows customization of connection settings, such as read/write deadlines and message handling.
Key Features of Gorilla WebSocket
1. Full WebSocket Support
Gorilla WebSocket fully implements the WebSocket protocol (RFC 6455), ensuring compatibility with WebSocket clients and servers.
2. Low-Level Control Over Connections
Developers can manage connection timeouts, buffers, and ping/pong messages, allowing fine-tuned control over WebSocket behavior.
3. Message Compression
Supports permessage-deflate, reducing bandwidth usage when sending large messages.
4. Secure Connections
Provides support for TLS (Transport Layer Security) to enable secure WebSocket communication.
5. Concurrent Read and Write Handling
Gorilla WebSocket allows concurrent reading and writing to connections, making it ideal for real-time applications.
6. Robust Error Handling
Built-in error-handling mechanisms ensure smooth operation, even in cases of network failures or unexpected disconnections.
Installing Gorilla WebSocket
To use Gorilla WebSocket in your Go project, install it using go get:
sh
CopyEdit
go get -u github.com/gorilla/websocket
How to Use Gorilla WebSocket in a Go Application
1. Creating a WebSocket Server in Go
Below is an example of a basic WebSocket server using Gorilla WebSocket:
go
CopyEdit
package main
import (
“fmt”
“net/http”
“github.com/gorilla/websocket”
)
// WebSocket upgrader
var upgrader = websocket.Upgrader{
CheckOrigin: func(r *http.Request) bool {
return true
},
}
func handleConnections(w http.ResponseWriter, r *http.Request) {
// Upgrade HTTP request to WebSocket
conn, err := upgrader.Upgrade(w, r, nil)
if err != nil {
fmt.Println(“Error upgrading to WebSocket:”, err)
return
}
defer conn.Close()
for {
// Read message from client
messageType, msg, err := conn.ReadMessage()
if err != nil {
fmt.Println(“Error reading message:”, err)
break
}
fmt.Println(“Received:”, string(msg))
// Echo message back to client
err = conn.WriteMessage(messageType, msg)
if err != nil {
fmt.Println(“Error writing message:”, err)
break
}
}
}
func main() {
http.HandleFunc(“/ws”, handleConnections)
fmt.Println(“WebSocket server started on :8080”)
err := http.ListenAndServe(“:8080”, nil)
if err != nil {
fmt.Println(“Server error:”, err)
}
}
2. Running the WebSocket Server
Save the above code as server.go and run:
sh
CopyEdit
go run server.go
The server will start at ws://localhost:8080/ws.
3. Creating a WebSocket Client in JavaScript
To connect a web browser client to the WebSocket server, use JavaScript:
js
CopyEdit
const socket = new WebSocket(“ws://localhost:8080/ws”);
socket.onopen = function () {
console.log(“Connected to server”);
socket.send(“Hello, Server!”);
};
socket.onmessage = function (event) {
console.log(“Received from server:”, event.data);
};
socket.onclose = function () {
console.log(“Connection closed”);
};
Advanced Features in Gorilla WebSocket
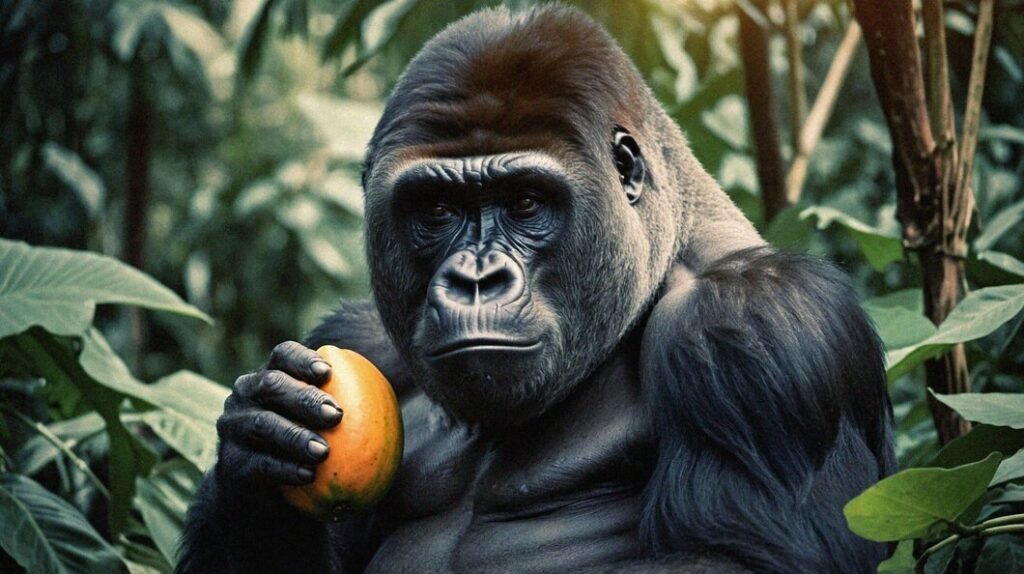
1. Handling Multiple Clients (Broadcasting Messages)
To support multiple clients, store connections in a map and send messages to all connected clients.
go
CopyEdit
var clients = make(map[*websocket.Conn]bool)
func handleConnections(w http.ResponseWriter, r *http.Request) {
conn, err := upgrader.Upgrade(w, r, nil)
if err != nil {
fmt.Println(“Error upgrading:”, err)
return
}
defer conn.Close()
clients[conn] = true
for {
_, msg, err := conn.ReadMessage()
if err != nil {
fmt.Println(“Error reading:”, err)
delete(clients, conn)
break
}
for client := range clients {
err := client.WriteMessage(websocket.TextMessage, msg)
if err != nil {
fmt.Println(“Error broadcasting:”, err)
client.Close()
delete(clients, client)
}
}
}
}
2. Setting Read and Write Deadlines
To prevent stale connections, set read and write deadlines:
go
CopyEdit
conn.SetReadDeadline(time.Now().Add(60 * time.Second))
conn.SetWriteDeadline(time.Now().Add(10 * time.Second))
3. Handling Ping/Pong Heartbeats
WebSockets use ping/pong messages to check if a connection is still active:
go
CopyEdit
conn.SetPongHandler(func(appData string) error {
fmt.Println(“Received pong”)
return nil
})
Use Cases of Gorilla WebSocket
Gorilla WebSocket is ideal for applications that require real-time updates:
- Chat applications (e.g., WhatsApp, Slack alternatives)
- Stock market dashboards (real-time price updates)
- Online gaming (instant multiplayer interactions)
- Live notifications (social media, messaging apps)
- IoT applications (real-time sensor data transmission)
Conclusion
Gorilla WebSocket is a powerful and efficient library for implementing WebSocket connections in Go applications. With features like message compression, concurrent handling, and secure communication, it is the go-to choice for developers building real-time web applications. By following the examples above, you can quickly set up a WebSocket server and client, ensuring smooth and reliable real-time communication in your Go projects.
FAQs
Is Gorilla WebSocket better than the standard Go WebSocket package?
Yes, Gorilla WebSocket offers more features, better error handling, and greater flexibility than the standard net/http WebSocket implementation.
Does Gorilla WebSocket support TLS encryption?
Yes, it supports secure WebSocket connections (wss://) using TLS.
Can Gorilla WebSocket handle multiple clients?
Yes, it allows handling multiple concurrent WebSocket connections efficiently.
Is Gorilla WebSocket suitable for production applications?
Absolutely! It is widely used in production for building real-time applications.
How do I close a WebSocket connection properly?
Use conn.Close() and ensure all resources are cleaned up properly when a connection closes.